***Definition:
A key is a value that you are looking for in
an array.
The simplest type of searching process is the sequential
search. In the sequential search, each element of the array is
compared to the key, in the order it appears in the array, until the first
element matching the key is found. If you are looking for an element that is near the front
of the array, the sequential search will find it quickly. The more data
that must be searched, the longer it will take to find the data that matches the
key using this process.
//sequential search
routine (non-function ... used in main)
#include <iostream.h>
#include <apvector.h>
int main(void)
{
apvector <int> array(10);
//"drudge"
filling the array
array[0]=20; array[1]=40; array[2]=100; array[3]=80; array[4]=10;
array[5]=60; array[6]=50; array[7]=90; array[8]=30; array[9]=70;
cout<< "Enter the number you want to find (from 10 to 100)…";
int key;
cin>> key;
int flag = 0;
// set flag to off
for(int i=0; i<10; i++)
// start to loop through the array
{
if (array[i] == key)
// if match is found
{
flag = 1; // turn flag on
break ; // break out of for loop
}
}
if (flag)
// if flag is TRUE (1)
{
cout<< "Your number is at
subscript position " << i <<".\n";
}
else
{
cout<< "Sorry, I could
not find your number in this array."<<endl<<endl;
}
return 0;
}
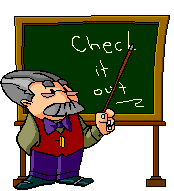 |
Now that we understand the elementary concept of the
sequential search, let us examine the sequential search used in a
function. While our example above worked for an array of 10
elements, our function will work for any size array -- a better
approach: |
//Sequential Search Function
//function receives the address of the array and the key to be found
int SequentialSearch (apvector <int> &array, int key)
{
int index = 0;
// set starting subscript to zero
while( (index < array.length( )) && (key != array[index]))
{
// loop while elements remain and key not found.
if (array[index] != key)
index++;
}
return (index); // if function returns a value less than array size,
// the value was found
}
|