Declaring Variables
All variables must be declared
before they can be used. |
How to declare a variable:
1. Choose the "type" you need.
2. Decide upon a name for the variable.
3. Use the following format for a declaration statement:
datatype variable identifier;
4. You may declare more than one variable of the same type by separating the variable names with commas.
int age, weight, height;
5. You can initialize a variable
(place a value into the variable location) in a declaration statement.
double mass = 3.45;
|
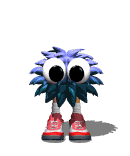 |
Variables that
are not initialized are NOT empty. If you do not initialize your variables, they will contain junk ("garbage") values left over from the program that last used the memory they occupy, until such time as the program places a value at that memory location. |
Constant Variables
Using const you can define variables whose values never change. You MUST
assign an initial value into a constant variable when it is
declared. If you do
not place this initial value, C++ will not allow you to assign a value
at a later time. Constants cannot be changed within a
program.
const int ageLimit
= 21; //this value
cannot be changed
|
C++ will allow you to declare a variable anywhere in the program as long as the variable is declared before you use it.
A good programming practice to develop is to declare variables at the top of a function.
Declaring variables in this manner makes for easier readability of the program. |
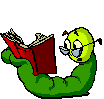 |
|

Return to Topic Menu |
Computer Science Main Page |
MathBits.com |
Terms of Use
|