We have seen the
advantages of using various methods of iteration, or looping.
Now
let's take a look at what happens when we combine looping
procedures.
The placing of one loop inside the body of another loop is
called nesting. When you "nest"
two loops, the outer loop
takes control of the number of complete repetitions of the inner
loop. While all
types of loops may be nested, the most
commonly nested loops are for
loops. |
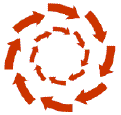
nested
loops
|
|
Let's look at an
example of nested loops at work.
We have all seen web page counters
that resemble the one shown below ( well, OK, maybe not quite this
spastic!!). Your car's odometer works in a similar manner.

This counter (if it
worked properly) and your car's odometer are little more than seven
or eight nested for
loops, each going from 0 to 9. The far-right number iterates the
fastest, visibly moving from 0 to 9 as you drive your car or increasing by one
as people visit a web site. A for
loop which imitates the movement of the far-right number is shown below:
|
for(num1 = 0; num1
<= 9; num1++)
{
cout << num1 << endl;
} |
The far-right number,
however, is not the
only number that is moving. All of the other numbers are moving also, but at a much slower
pace. For every 10 numbers that move in the column on the right, the adjacent
column is incremented by one. The two nested loops shown below may be
used to imitate the movement of the two far-right numbers of a web counter or an odometer:
The number of digits in the web
page counter or the odometer determine the number of
nested loops needed to imitate the process.
When
working with nested loops, the outer loop changes
only after the inner loop is completely finished (or is interrupted.). |
Let's take a look at a trace of two
nested loops. In order to keep the trace manageable, the
number of iterations have been
shortened.
for(num2 = 0; num2 <=
3; num2++)
{
for(num1 = 0; num1 <= 2; num1++)
{
cout<< num2<< " " << num1<<
endl;
}
} |
Memory |
Screen |
int
num2 |
int
num1 |
|
0 |
|
0 |
|
|
|
1 |
|
|
|
2 |
|
|
|
3
end loop |
|
1 |
|
0 |
|
|
|
1 |
|
|
|
2 |
|
|
|
3
end loop |
|
2 |
|
0 |
|
|
|
1 |
|
|
|
2 |
|
|
|
3
end loop |
|
3 |
|
0 |
|
|
|
1 |
|
|
|
2 |
|
|
|
3
end loop |
|
4
end loop |
|
|
Remember,
in the memory, for
loops will register a value one beyond (or the step beyond)
the requested ending value in order to disengage the loop. |
0
0
0 1
0 2
1 0
1 1
1 2
2 0
2 1
2 2
3 0
3 1
3 2 |
Are
we getting a little loopy?
|
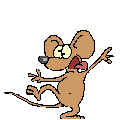 |
|