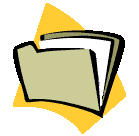 |
A file is a container for data. Think of a
file as a drawer in a file cabinet which needs to be opened and
closed. Before you can put something into a file, or take
something out, you must open the file (the drawer). When you are finished
using the file, the file (the drawer) must be closed. |
Steps to create (or write to) a sequential access
file:
1. Declare a stream variable name:
ofstream
fout; |
//each file has its own stream buffer |
ofstream
is short for output file stream
fout is
the stream variable name
(and may be any legal C++ variable name.)
Naming the stream variable "fout" is
helpful in remembering
that the information is going "out" to the file.
2. Open the file:
fout.open("scores.dat",
ios::out);
fout
is the stream variable name previously declared
"scores.dat"
is the name of the file
ios::out
is the steam operation mode
(your compiler may not require that you specify
the stream operation mode.)
3. Write data to the file:
fout<<grade<<endl;
fout<<"Mr. Spock\n";
The data must be separated with
space characters or end-of-line characters (carriage return), or the data will
run together in the file and be unreadable. Try to save the data to the
file in the same manner that you would display it on the screen.
If the iomanip.h
header file is used, you will be able to use familiar formatting commands with
file output.
fout<<setprecision(2);
fout<<setw(10)<<3.14159;
4. Close the file:
fout.close( );
Closing the file writes any data
remaining in the buffer to the file, releases the file from the program, and
updates the file directory to reflect the file's new size. As soon as your
program is finished accessing the file, the file should be closed. Most
systems close any data files when a program terminates. Should data remain
in the buffer when the program terminates, you may loose that data. Don't
take the chance --- close the file!
|