Return to Topic Menu
|
Computer Science Main Page |
MathBits.com
|
Terms of Use
|
Resource CD
Working with Multiple
Files
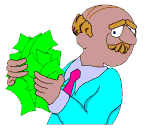 |
It is possible to open more
than one file at a time. Simply declare and use a
separate stream variable name (fout, fin, fout2, fin2 --
file pointer) for each file. You may need to use this
multiple file process for situations where you need to
compare file data, to use one set of file data to create a
new set of adjusted data, or to simply use a combination of
various files to accomplish your programming task. |
EXAMPLE:
//Retrieve all of the zipcodes that
are 13090 from an existing file which
//contains name, address and zipcode as three separate
entries.
//Place the newly found names, addresses and zipcodes into a
new file.
#include <iostream.h>
#include <fstream.h>
#include "apstring.cpp"
#include <stdlib.h>
int main(void)
{
apstring name, address, dummy;
int zipcode;
ifstream fin;
ofstream fout;
system("CLS");
fin.open("add.dat", ios::in);
assert(! fin.fail( ) );
fout.open("newZip.dat", ios::out);
assert (! fout.fail( ) );
getline(fin,name);
//priming loop
getline(fin,address);
fin>>zipcode;
getline(fin,dummy);
while(!fin.eof( ) )
{
if (zipcode==13090)
{
fout<<name<<endl;
fout<<address<<endl;
fout<<zipcode<<endl;
}
getline(fin,name);
getline(fin,address);
fin>>zipcode;
getline(fin,dummy);
}
fout.close();
fin.close();
return 0;
}
If you need to
open two or more files for input, simply give
the stream variables different names, such as
fin, fin2, fin3, etc. |
|